Step 4: Create Extension Project
In this tutorial, you will create a ThingWorx extension that retrieves weather information using OpenWeatherMap API.
Create Account
In this part of the lesson, you will create a free account in OpenWeatherMap that creates an AppKey so you can access their REST API.
- Sign-up for a free account.
- Log in to your account.
- Create a new API Key
NOTE: We will use this generated API key as a parameter in the REST calls.
Create New Extension Project
NOTE: Make sure that you are in the ThingWorx Extension Perspective. To verify, you should see a plus icon:

in the menu bar. If you don’t see this, you are probably in the wrong perspective. Go back to the previous step to learn how to set the perspective to ThingWorx Extension in Eclipse.
- Go to File->New->Project.
- Click ThingWorx->ThingWorx Extension Project.
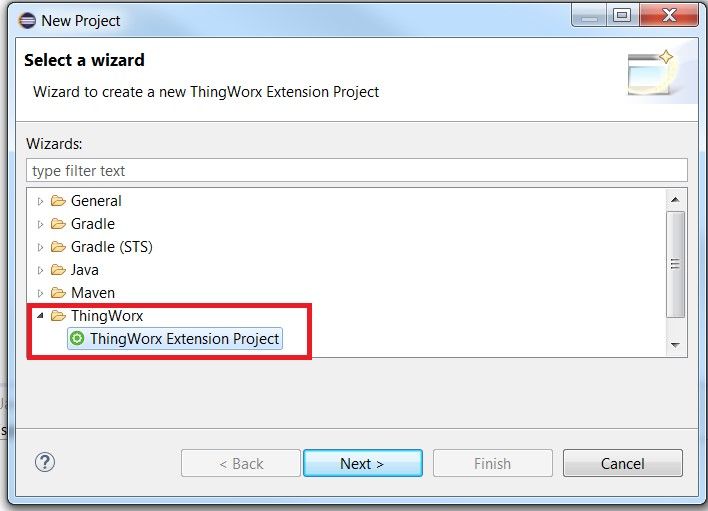
Click Next.
NOTE: A New ThingWorx Extension window will appear.
- Enter the Project Name (for example, MyThingworxWeatherExtension).
- Select Gradle or Ant as your build framework.
- Enter the SDK location by browsing to the directory where the Extension SDK is storeed.
NOTE: The Eclipse Plugin accepts the Extension SDK version 6.6 or higher.
- Enter the Vendor information (for example, ThingWorx Labs).
- Change the default package version from 1.0.0 to support extension dependency.
NOTE: The information from ThingWorx Extension Properties is used to populate the metadata.xml file in your project. The metadata.xml file contains information about the extension and details for the various artifacts within the extension. The information in this file is used in the import process in ThingWorx to create and initialize the entities.
- Select the JRE version to 1.8.
- Click Next then click Finish. Your newly created project is added to the Package Explorer tab.
Create New Entity
- Select your project and click Add to create a new entity.

NOTE: You can also access this from the ThingWorx menu on the menu bar.
- Create a Thing Template for your MyThingWorxWeatherExtension Project.
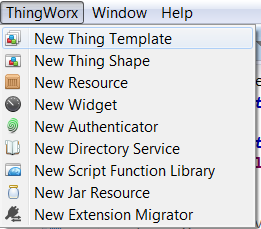
NOTE: In this guide, we are using a Template, but in a real-world scenario, you may consider using a Thing Shape to encapsulate extension functionality. By using Thing Shapes you give users of your extension the ability to easily add new functionality to existing Things. It is simple to add a new Thing Shape to an existing Thing Template, while using the properties or services defined by a Thing Template would require recreating all existing assets using the new Template. Since subscriptions cannot be created on Thing Shapes, you might choose to create Thing Templates that implement one or more subscriptions for convenience.
- In the pop-up window, browse to add the source folder of your project in Source Folder.
NOTE: It should default to the src directory of your project. In our case it will be MyThingworxWeatherExtension/src.
- Browse to add the package where you want to create this new class, or simply give it a name (such as com.thingworx.weather).
- Enter a name and description to your Thing Template (WeatherThingTemplate).
NOTE: By default, the Base Thing Template is set to GenericThing.
- Select Next.
NOTE: If you want to give other users of this entity permission to edit it in ThingWorx Composer, select the entity as an editable entity. Only non-editable entities can be upgraded in place; editable entities must be deleted and recreated when your extension is updated. If you need to make it possible to customize the extension, consider using a configuration table to save user customizations.
- Select Finish.
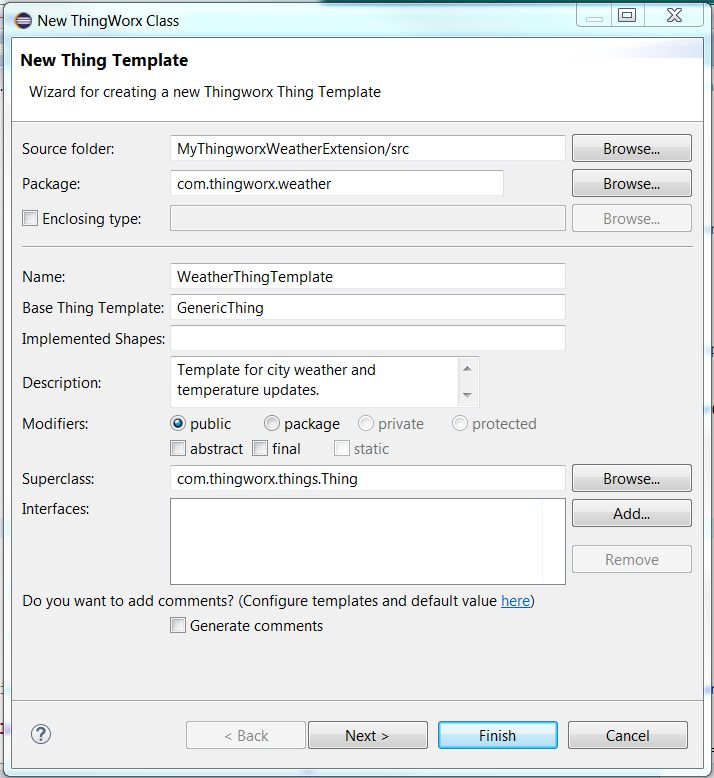
- Verify that you have a WeatherThingTemplate class created that extends the Thing class.
@ThingworxBaseTemplateDefinition(name = "GenericThing")
public class WeatherThingTemplate extends Thing {
public WeatherThingTemplate() {
// TODO Auto-generated constructor stub
}
}
NOTE: You might see a warning to add a serial version. You can add a default or generated serial value.
Step 5: Add Properties
In this section, you are going to add CurrentCity, Temperature and WeatherDescription properties to the WeatherThingTemplate. These properties are associated with the Thing Template and add the @ThingworxPropertyDefinitions annotation before the class definition in the code.
- Right click inside the WeatherThingTemplate class or right click on the WeatherThingTemplate class from the Package Explorer.
- Select ThingWorx Source-> Add Property.
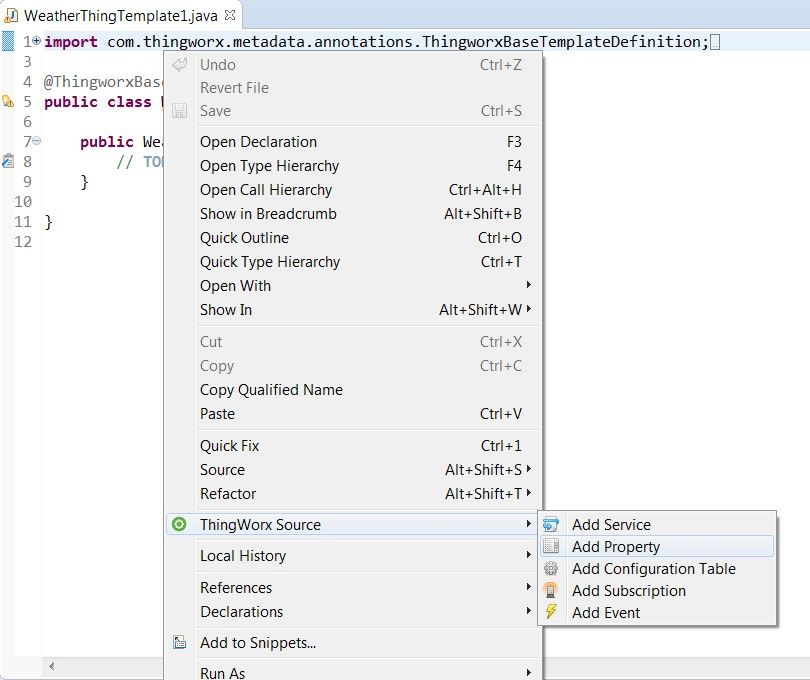
In the popup window, create a property to store the city name.
- Name = CurrentCity, Base Type = STRING, Description = ‘’
- Select the Has Default Value checkbox and enter a city name (eg. Boston). This will be the default value unless a specific value is passed.
- Select the “Persistent” checkbox. This will maintain the property value if a system restart occurs. NOTE: If you select the Logged checkbox, the property value is logged to a data store. If you select the Read Only checkbox, the data will be static.
- Select VALUE from the Data Change Type drop down menu
NOTE: This allows any Thing in the system to subscribe to a data change event for this property.
- Choose to use one of the following Data Change Types:
Data Change | Type Description |
Always | Fires the event to subscribers for any property value change |
Never | Does not fire a change event |
On | For most values, any change will trigger this. |
Off | Fires the event if the new value is false |
Value | For numbers, if the new value has changed by more than the threshold value, fire the change event. For non-numbers, this setting behaves the same as Always. |
- Select Finish.
- Create another property called Temperature with a base type of NUMBER. You can keep the default values for the other parameters.
- Create another property called WeatherDescription with a base type of STRING. Keep the default values for the other parameters.
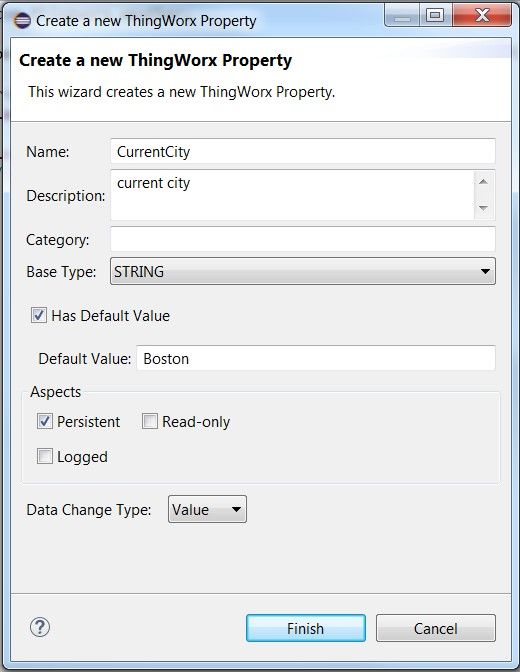
Step 6: Create Configuration Table
In this part of the lesson, we will create a configuration table to store the API Id that you generated from the openMapsWeather. Configuration tables are used for Thing Templates to store values similar to properties that do not change often.
- Right-click inside the WeatherThingTemplate class and select ThingWorx Source->Add Configuration Table.
- Create a new configuration table with name OpenWeatherMapConfigurationTable.
- Click Add in the Data Shape Field Definitions frame.
NOTE: Configuration tables require fields (columns) with a defined table structure (DataShape).
- Enter appid as the name with a base type STRING.
- Select the Required checkbox.
- Click OK, then Finish to add the Configuration Table
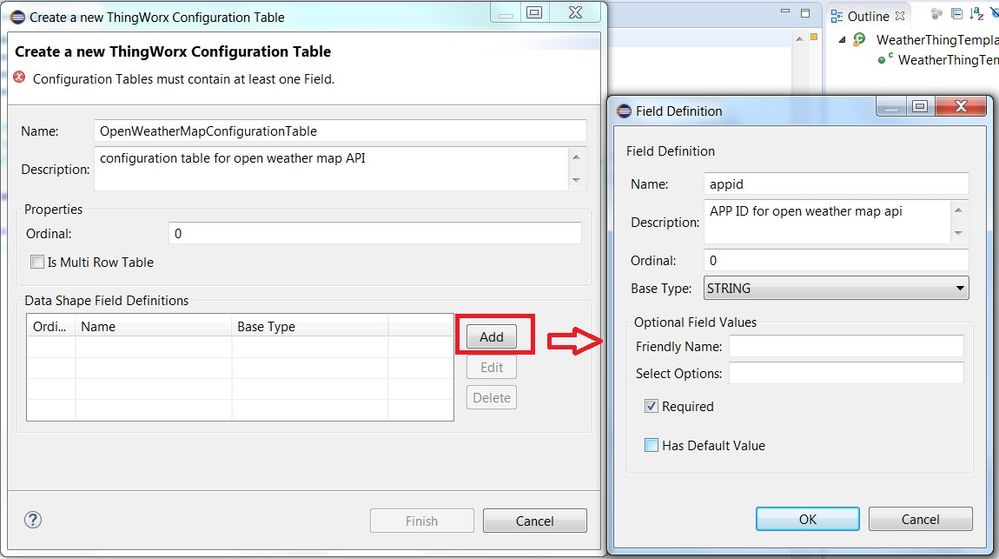
- To use the appid in the REST calls, you need to obtain the value from the configuration table and assign it to a field variable in the Java code. We will use the initializeThing method to obtain the appid value at runtime.
NOTE: The initializeThing() method acts as an initialization hook for the Thing. Every time a Thing is created or modified, this method is executed and the value of appid is obtained from the configuration table and stored in a global field variable of the class. initializeThing() must call super.initializeThing() to ensure it performs initialization of the Thing.
- Create the initializeThing() method and field variable _appid with base type STRING anywhere in the WeatherThingTemplate class.
private static Logger _logger = LogUtilities.getInstance().getApplicationLogger(WeatherThingTemplate.class);
private String _appid;
@Override
public void initializeThing() throws Exception {
super.initializeThing();
_appid = (String) this.getConfigurationSetting("OpenWeatherMapConfigurationTable", "appid");
}
NOTE: In the code above we used ThingWorx LogUtilities to get a reference to the ThingWorx logging system, then assigned the reference to the variable _logger. In the steps below we will use this variable to log information. There are multiple kinds of loggers and log levels used in the ThingWorx Platform, but we recommend that you use the application or script loggers for logging anything from inside extension services. If prompted to import the logger, use slf4j.
Click here to view Part 3 of this guide.